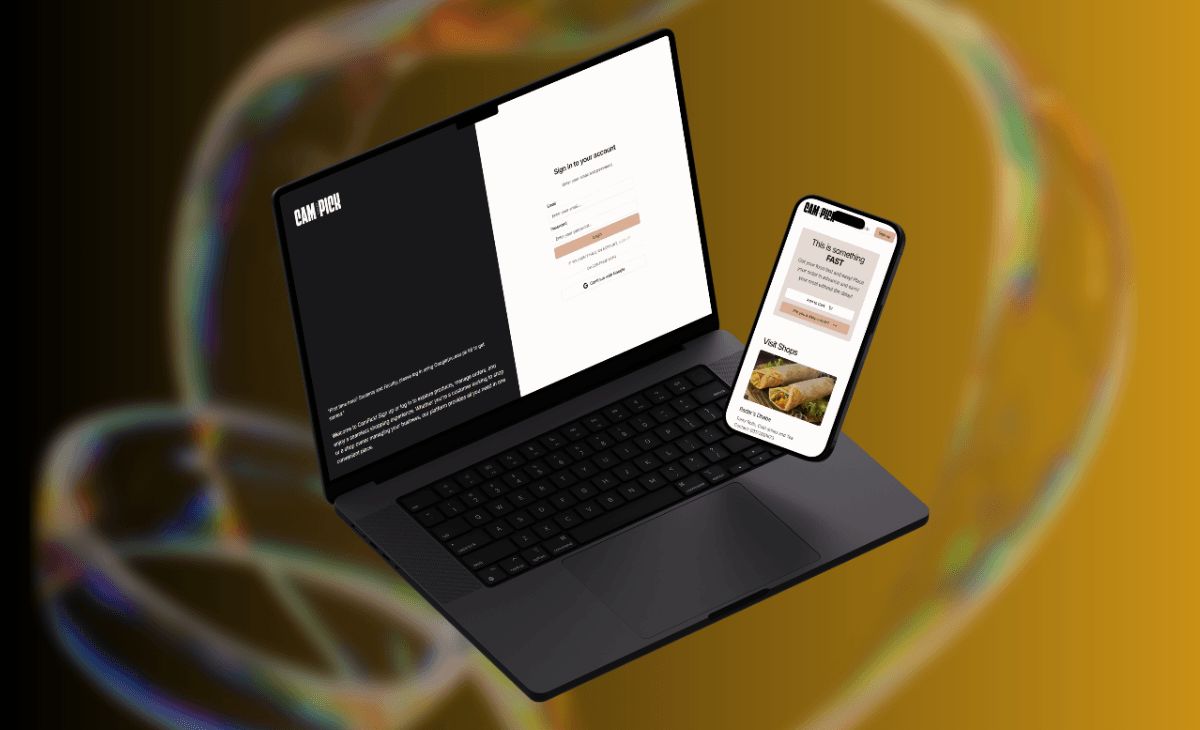
CamPick NUCES - Campus Food Ordering System
Introduction
CamPick NUCES is a cutting-edge food ordering and management platform designed specifically for university campuses. It aims to simplify the food ordering process for students while providing shop owners with robust management tools.
Technology Stack
Backend
- Framework: Node.js with Express.js for RESTful APIs
- Database: MySQL for relational database management
- Real-time Communication: Socket.IO
Frontend
- Framework: React.js
Authentication
- Method: JWT for secure token-based authentication
- OAuth: Passport.js for Google OAuth integration
Cloud Services
- Image Management: Cloudinary
- Notifications: SMTP for email services
Development Tools
- Containerization: Docker
- Version Control: Git
Key Features
1. Authentication
- Multi-role authentication (students, teachers, shop owners)
- Google OAuth integration
- Email verification and OTP system
2. User Management
- User profiles with role-based access control
- Alert system for student behavior monitoring
- Administrative oversight
3. Order Management
- Real-time order tracking
- Create, update, and delete orders
- Order integrity validation
4. Shop Management
- Shop creation and performance analytics
- Owner dashboard
- Communication and payment integration
5. Menu Management
- Dynamic menu item creation
- Shop-specific menu associations
- Detailed item descriptions and pricing
6. Payment Management
- Multiple payment methods support
- Payment verification system
- Automated revenue tracking
Business Logic
Core Principles
- User Alerts: Three-strike policy for accountability
- Order Processing: Validation of item availability and user eligibility
- Revenue Management: Automated calculations and real-time updates
- Audit Logging: Comprehensive action tracking
Folder Structure
CamPick NUCES Project
│
└── src
├── controllers
│ ├── authController.js # Manages user authentication (login, registration, etc.)
│ ├── userController.js # Handles user profile management and related operations
│ ├── shopController.js # Manages shop-related functionalities (creation, updates, etc.)
│ ├── menuController.js # Handles menu item management for shops
│ ├── orderController.js # Manages order processing, tracking, and history
│ ├── paymentController.js # Handles payment processing and verification
│ ├── googleAuthController.js # Manages Google OAuth authentication
│ ├── nlQueryController.js # Handles natural language queries (if applicable)
│ └── upload.js # Manages file uploads (e.g., images, documents)
│
├── models
│ ├── user_schema.sql # SQL script for creating the users table and related structures
│ ├── shop_schema.sql # SQL script for creating the shops table and related structures
│ ├── order_schema.sql # SQL script for creating the orders table and related structures
│ ├── payment_schema.sql # SQL script for creating the payments table and related structures
│ └── menu_schema.sql # SQL script for creating the menu items table and related structures
│
├── routes
│ └── routes.js # Main routing file that connects controllers to specific endpoints
│
├── utils
│ ├── generateId.js # Utility for generating unique IDs (e.g., UUIDs)
│ ├── emailTemplate.js # Functions for generating email templates (e.g., order confirmations)
│ ├── cloudinary.js # Configuration and utility functions for Cloudinary image management
│ ├── shopUtils.js # Utility functions related to shop operations (e.g., analytics, sales)
│ ├── orderUtils.js # Utility functions for order processing and validation
│ ├── paymentUtils.js # Utility functions for payment processing and verification
│ └── userUtils.js # Utility functions for user management and validation
│
├── middleware
│ └── middleware.js # Custom middleware for authentication, logging, etc.
│
├── config
│ ├── database.js # Database connection configuration and setup
│ └── server.js # Server configuration and initialization
│
├── demo
│ └── demo.js # Sample data generation and testing scripts
│
├── app.js # Main application file that initializes the server and middleware
├── package.json # Project metadata and dependencies
├── .env # Environment variables for configuration (e.g., database credentials, API keys)
└── README.md # Documentation for setting up and using the project
Database Schema
Tables
- Users: User information, roles, verification status
- Shops: Shop details, owner, revenue
- Menu Items: Available order items, shop-linked
- Orders: Order placement details
- Payments: Payment information and statuses
- Audit Logs: Changes tracking for accountability
Benefits
For Students
- Simplified food ordering
- Real-time updates
- Secure payment options
For Shop Owners
- Effective business management
- Sales tracking
- Customer behavior insights
For Administration
- Student behavior monitoring
- Policy compliance
- Transparent dining operations overview
For the Community
- Enhanced campus dining experience
- Convenient and efficient food delivery service
Conclusion
CamPick NUCES demonstrates the integration of modern web technologies to address campus dining challenges, prioritizing convenience, security, and efficiency for all users.